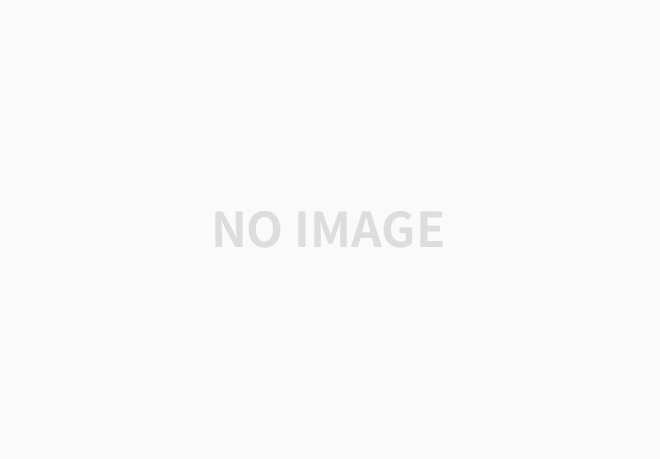
Like if-else, the switch produces a result or runs a certain code based on conditions. Switch provides a better readability compared to if-else. Let's see the syntax of the switch in Java
List of content
Syntax
switch(<Value>)
Take a value that will be compared in the case line. There type can be used and the possible type list is as follows (other types such as double cannot be used)
byte, short, int, char, Byte, Short, Integer, Character, String, enum
case <condition>:
Sets a condition to match the value provided
default :
Sets the default value that will be used when none of the above cases matches
break
Stop the switch code. Without this even if a value matches a condition the code will run until the end (fall through)
Examples
There are two main ways to use the switch in Java, traditional and enhanced which is available for Java 14 and above
Switch
▶ With No Return
switch (number) {
case 0:
System.out.println("ZERO");
break;
case 1:
System.out.println("ONE");
break;
case 2:
System.out.println("TWO");
break;
case 3:
System.out.println("THREE");
break;
default:
System.out.println("OTHER");
break;
}
▶ With No Return (Multi Conditions)
switch (number) {
case 0:
System.out.println("ZERO");
break;
case 1:
System.out.println("ONE");
break;
case 2: case 3:
System.out.println("TWO");
System.out.println("THREE");
break;
default:
System.out.println("OTHER");
break;
}
▶ With Return
switch (number) {
case 0:
return "ZERO";
break;
case 1:
return "ONE";
break;
case 2: case 3:
return "TWO THREE";
break;
default:
return "OTHER";
break;
}
Enhanced Switch
자바 14 이후로 사용가능한 기능으로 이전 스위치에 비해 문법이 간결함
▶ With No Return
switch (value) {
case "1" -> System.out.println("good");
case "2" -> System.out.println("bad");
default -> System.out.println("What");
}
▶ With No Return (Multi Conditions)
switch (value) {
case "1", "2" -> System.out.println("good");
default -> System.out.println("What");
}
▶ With Return
switch (value) {
case "1" -> {
System.out.println("good");
return "1";
}
case "2" -> {
System.out.println("bad");
return "2";
}
default -> {
System.out.println("What");
return "3";
}
}
Or
return switch (value) {
case "1" -> "1";
case "2" -> "2";
default -> "3";
}
So far we have explored the switch in Java.
'Backend > Java' 카테고리의 다른 글
Inheritance (0) | 2023.12.28 |
---|---|
Class (0) | 2023.12.28 |
Spring Boot Form (10) | 2023.10.28 |
Spring Beans - Dependency Injection (1) | 2023.10.07 |
Spring Boot Security (1) | 2023.10.01 |