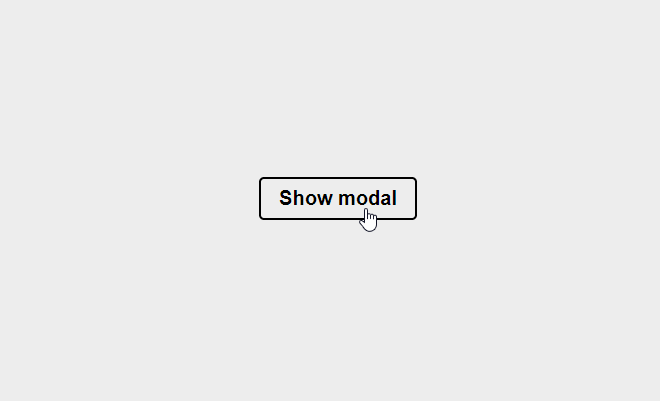
A modal in web programming refers to a popup or a dialog box that appears on top of the page to interact with users. Modal is a great way to get users’ attention. Let’s see how to implement is with HTML, CSS and JavaScript
Implementation
First, we need a modal in the HTML. In this example, I kept the code as simple as possible so that we can focus on the topic.
In the HTML, create a div and add a form with classes to style them later in the CSS file
<!-- index.html -->
<body>
<button id="auth">auth</button>
<div class="modal">
<form>
<i id="close">❌</i>
</form>
</div>
</body>
To make the modal element to be out of the flow we can either use position: absolute; or position: fixed; I prefer to use position: fixed; because this always covers the whole screen regardless of the content size. Hide the element with the property of your choice, I used display:none; here for the simplicity.
.modal {
height: 100vh;
width: 100vw;
position: fixed;
top: 0;
left: 0;
background-color: rgba(0, 0, 0, .3);
display: none;
align-items: center;
justify-content: center;
}
form {
height: 300px;
width: 300px;
background-color: aliceblue;
position: relative;
}
i {
position: absolute;
top: 5px;
right: 10px;
cursor: pointer;
}
For JavaScript, implement click events as follow. Then it is done!
const auth = document.getElementById('auth')
const modal = document.querySelector('.modal')
const close = document.getElementById('close')
// opens modal
auth.addEventListener('click', () => {
modal.style.display = 'flex'
})
// closes modal when X icon is clicked
close.addEventListener('click', () => {
modal.style.display = 'none'
})
// closes modal when anywhere outside the modal form is clicked
modal.addEventListener('click', (e) => {
modal.style.display = 'none'
})
There are many ways to implement the same feature and I showed you one of the ways that I use often. I prefer using a modal instead of creating a separate page whenever possible for the simplicity and clarity it provides.
References
How To Make a Modal Box With CSS and JavaScript
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
'Frontend > JavaScript' 카테고리의 다른 글
Data Request (1) | 2023.03.24 |
---|---|
Day / Night Mode with JavaScript (1) | 2023.03.24 |
How to Tell PC from Mobile in A Responsive APP (1) | 2023.03.23 |
Infinite Scrolling (0) | 2023.03.14 |
JS Component - Expanding Search Bar (1) | 2023.03.08 |