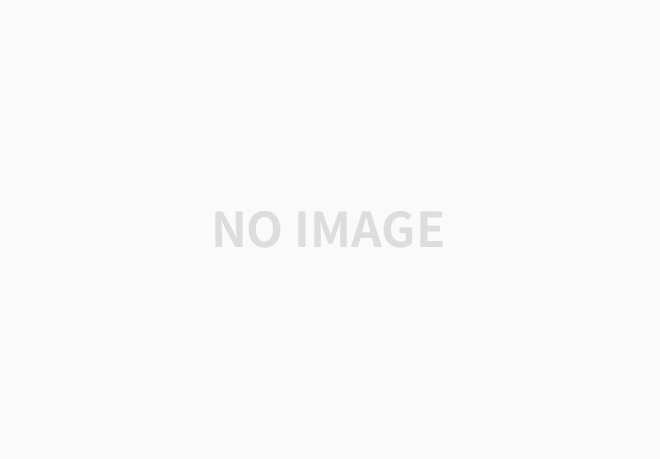
The decorator in TypeScript is a feature that enables us to add meta data or business logic without having to change the orginal code format.
For example, when we have multiple classes with the same attribute name, type, with the same value, we can use the decorator to reduce redundancy
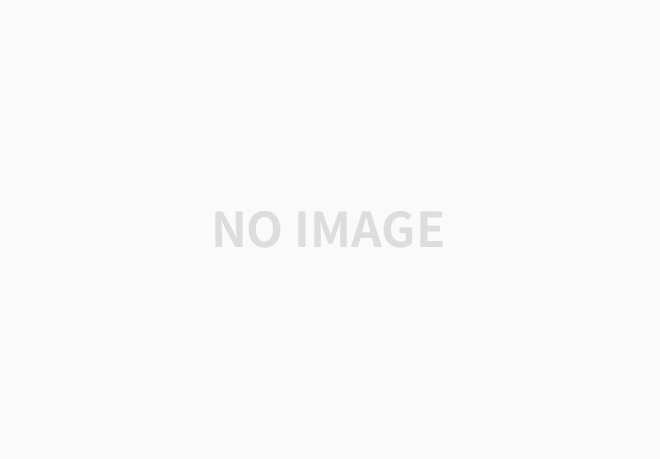
How to Use It?
This feature is in test at the moment, so we need a set up to use this. Open the console and run the command shown below.
npx tsc --init
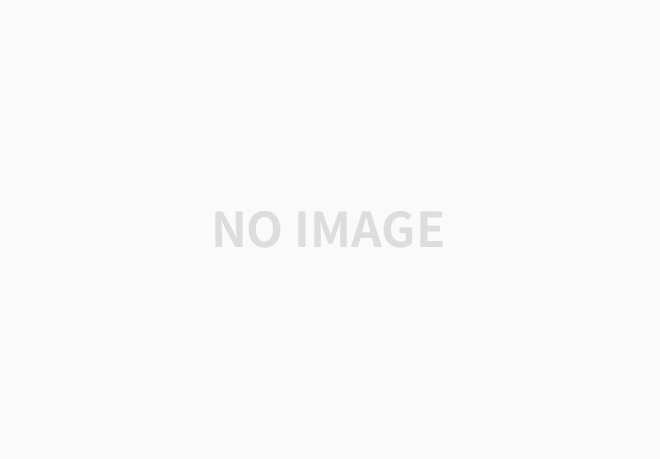
After running, a file called 'tsconfig.json' will be created. There are many options written in the file but we don't need any of them so if you want you can delete them all.
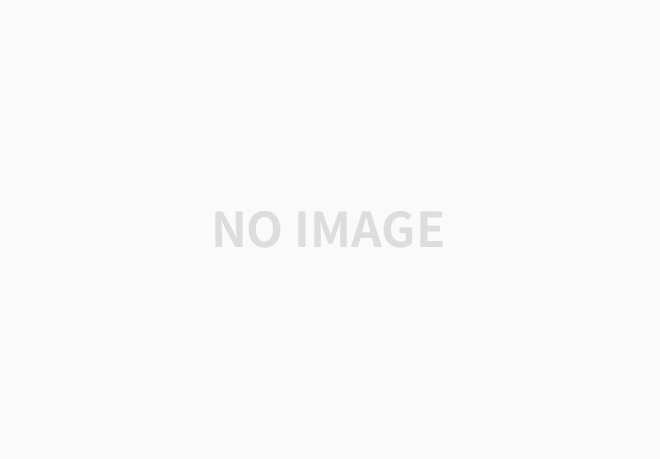
To use the decorator add the option shown below
{
"compilerOptions": {
"experimentalDecorators": true
}
}
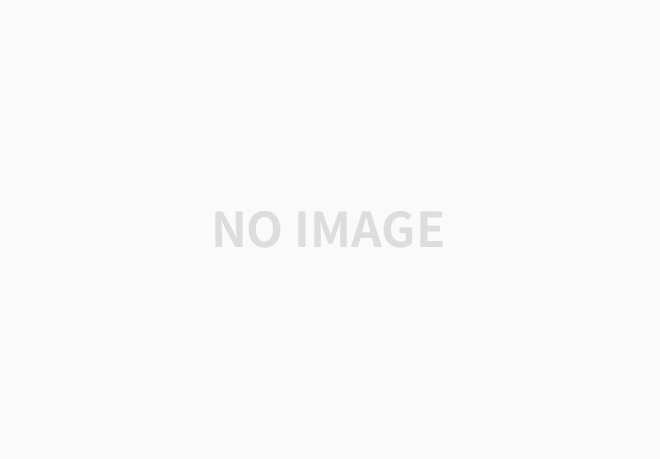
Go to the code file and create a decorator (basically it is a function with a function as a parameter). Add the decorator to the classes
function DecoTest(type:Function) {
type.prototype.type = 'number'
}
@DecoTest
class ClassOne {
type: string = 'number'
}
@DecoTest
class ClassTwo {
type: string = 'number'
}
console.log(new ClassOne().type)
console.log(new ClassTwo().type)
Compile the file
npx tsc

You will see that we can set up the types for both the classes without having to change them separately

Adding Parameters
But the thing is it is not possible to change the value dinamically.
We can add another function to dinamically change the values for each class. Update the code as shown below.
function DecoTest(type:number) { // outer function -> gets parameters
return (output: Function) => { // inner function -> returns decorator
output.prototype.value = type
}
}
@DecoTest(1)
class ClassOne {
type: string = ''
value: number
}
@DecoTest(2)
class ClassTwo {
type: string = ''
value: number
}
Complie the file
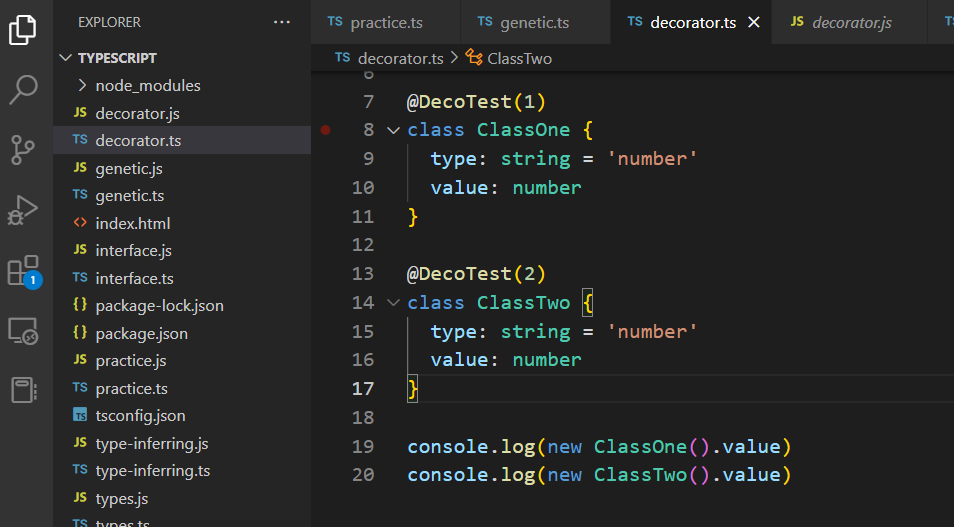
Then you will see that each class returns a value that is passed as a parameter in the decorator annotation.
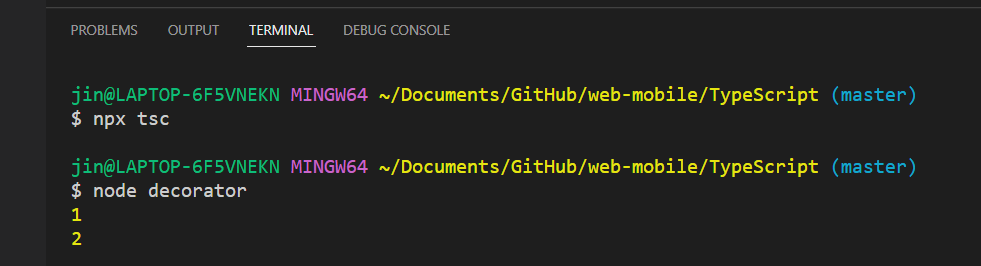
In this writing, we have seen how to use decorators in TypeScript.
Source Code
https://github.com/jin-co/web-mobile/tree/master/TypeScript
GitHub - jin-co/web-mobile
Contribute to jin-co/web-mobile development by creating an account on GitHub.
github.com
References
https://www.typescriptlang.org/tsconfig
TSConfig Reference - Docs on every TSConfig option
From allowJs to useDefineForClassFields the TSConfig reference includes information about all of the active compiler flags setting up a TypeScript project.
www.typescriptlang.org
'Frontend > TypeScript' 카테고리의 다른 글
Generic (9) | 2023.05.12 |
---|---|
Interface (0) | 2023.03.06 |
TypeScript (0) | 2023.03.06 |