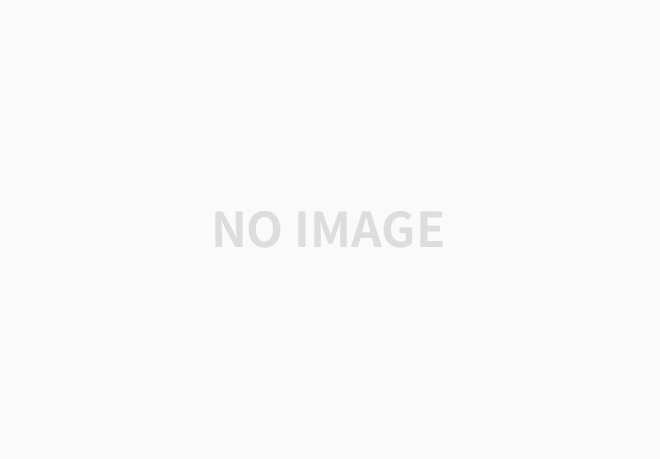
In Object Oriented Programming, entities are used to pass data. Entities often have fields that specify the characteristics of the entity. It is best practice to use getters and setters to prevent the fields from getting changed directly but the problem is that the getters and setters are boilerplate codes that have nothing to do with the business logic to which effort should be put. Lombok is a dependency that creates the boilerplate codes automatically using annotations and in this writing, we will see how to use it.
List of Contents
Implementation
We will use the below entity for the demonstration
public class Employee {
private String firstName;
private String lastName;
private String address;
public Employee() {
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
Dependency
We can either add it when creating a project
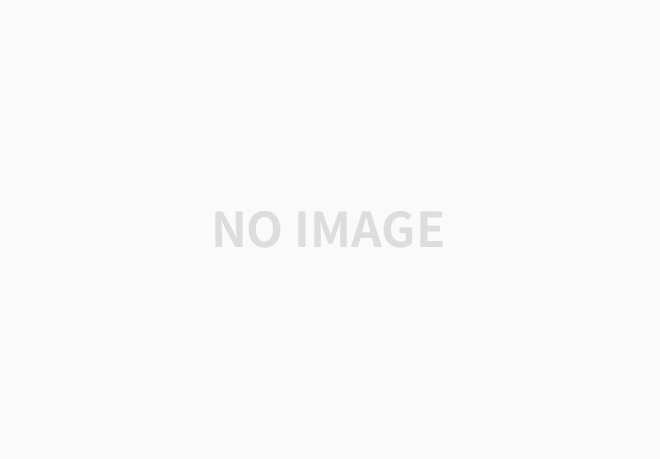
or open the pom.xml file and add the below code in the dependencies
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
After adding the dependency add the annotations above the entity class
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
We can check if the annotation is working by adding the annotation and leaving the code that does the same function
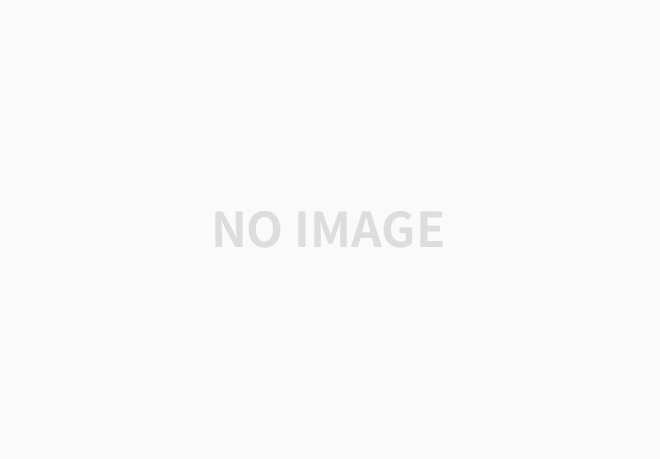
Code
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
public class Employee {
private String firstName;
private String lastName;
private String address;
}
In this writing, we have seen how we can use Lombok to remove boilerplate codes.
'Backend > Java' 카테고리의 다른 글
Method Overriding VS Overloading (1) | 2023.12.31 |
---|---|
java: error: release version 21 not supported (0) | 2023.12.30 |
Inheritance (0) | 2023.12.28 |
Class (0) | 2023.12.28 |
Switch (0) | 2023.12.26 |